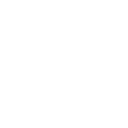
Adding trees via code
Hi Aaron and thanks for a great product.
I have loads of trees on maps, since I have "forests" in the game title, and when I realized the frame rate I'm getting with normal models, I searched for some optimized solution and found LushLOD trees on Asset store.
Your instructions on how to add them on a static map are great, and thank you for that, and for the all the comments in the code.
In my game, I want to add trees via my code (c#), since I'm generating a random world map. Voxel world, I set trees locations int x, y, z via noise map. Can you help out with that kind of LushLOD trees usage? :-)
I can reverse engineer your code to find out how to use it via code and insert into my game, but it'll take time, so I'd be really happy if you could write a small tutorial (doesn't need to be detailed as the other ones).
Thanks again and good luck
Customer support service by UserEcho
Sure I can write a tutorial for you. But for the time being, I'll just tell you real quick.
Adding trees while the game is running works just the same as adding any other prefab to your scene while the game is running, with ONE EXCEPTION...
LushLOD Trees have a system I called "parents". The parenting of the trees is basically this... each tree is linked to the trees around it. And essentially, one will be a "parent", and the other trees around it will be "children". When the camera is very close to the trees, then ALL of the trees that are close to the camera will measure their distance to the camera at frequent intervals, so that they can transition from billboards as necessary.
However, when the trees are far away from the camera, it would be excessive for ALL of them to measure their distance to the camera... as that would be expensive on the processor, especially for thousands of trees. That's what the parents are for. Basically, when the trees are far away, ONLY the parent is active, and ONLY the parent's Update() function will run, and ONLY the parent will measure its distance to the camera (at a less frequent interval too). Then, if the parent detects that the camera is getting close, the parent will "awaken" all of its children, and all the child trees (the trees that are nearby that parent tree) will then start to measure their distance to the camera, so that they can transition from billboards as the camera approaches closer. Hope that makes sense.
Well anyway, so here's what this means for adding trees while the game is running:
1) Anytime you add, delete, or even move a LushLOD Tree while the game is running, the parents need to be fully recalculated.
If I remember correctly, I set it up so that if you add, delete, or move a tree, it will recalculate parents automatically. A warning will appear in the console the first time this happens. Recalculating parents for thousands of trees is very slow, and if it happens after EVERY tree you add, then that's thousands of times that parents will be recalculated.
So basically, all of this was to say, that you need to make sure it doesn't recalculate parents more than once. What you want to do is add all the trees (while the game is running), and then after you're done adding all the trees, then recalculate the parents ONCE. But don't let it recalculate parents after each tree you add. Just do the parents calculation once, after each time you update the trees in the scene.
Okay so in the _LushLODTreesManager.cs script, you'll see a function called something like RecalculateParents(). That's the function that calculates the parents. You basically need to add some of your own code to that function, to PREVENT that function from being run multiple times. Some code at the top of that function which will check to see if your custom code (the code you wrote to add trees to your scene) is DONE making all your changes to the trees. And if you're not done, then just tell it to exit that RecalculateParents() function. Don't let it run the RecalculateParents() over and over again after every tree you update, because that will make it take forever to update your tree. And then, when your custom code is finished add trees to the scene, then you'll just want to manually call the RecalculateParents() function, and let it recalculate the parents. And that's about it, your scene should be good to go.
One disclaimer: It's been a long time since I wrote the RecalculateParents() function. It's possible, I may have actually added some code to let you control when and if it recalculates the parents. I don't *think* I added any such code, and I'm *pretty sure* it will try to recalculate parents after every tree you add to the scene. However, I may have added a delay of a few seconds, so that it'll wait a few seconds before recalculating parents... allowing you some time to add multiple trees before a recalculation of parents occurs. But either way, you'll still want to take direct control of RecalculateParents(), to ensure that function is only called once, after you are fully done.
Let me know if you run into any problems I didn't address above. I may be forgetting some key step, it's been a while since I played around with adding LushLOD Trees to a scene dynamically.
Thank you so much for such a quick answer.
I was thinking about parents (not mine, but tree parents), and I think my situation is simpler than "yours". As every voxel world, I work with chunks (8*8*8), so I can simply set chunks as parents. Any change on trees in chunk, parent is easy to find and notify.
But I think chunk is too small of a measure. Trees are usually 5-10m apart, so there will be about 2 per chunk.
Maybe I should have 1 parent tree per 8 chunks... What's the recommended maximum distance between the parent and a child tree, so it would still look smooth?
So, I need to:
add LushLODTreesManager to the scene,
add TreesRoot,
use your prefabs using my instantiate script and add them as children of TreesRoot,
tell some tree or other game object that it has these trees as children,
add two scripts to the camera,
rework your settings canvas into my "trees settings" so players can set things by themselves
Thanks again,
Karlo
The parents can't have children too far away. The way I designed it is, the distance that the parents can handle is directly tired to the LOD distance you set in the manager. When you set the LOD distance high, then the trees start to transition much further away from the camera... which means the parents can handle children further away from the parent. But when the LOD distance is very small, then the parents also have a very small range for their children, which also increases the number of parents required in the scene.
Reason for this is, if I remember correctly, if you had a huge distance on the parents from their children, but your LOD distance was set really low, then the parent would either turn on some of its children really early (long before you are close enough that those children need to transition, OR... the parent wouldn't turn them on until they should have already began their transition. Something like that is the problem. So the parent distance is tied to the LOD distance.